In web development, especially when working with PHP-based frameworks like Magento, Laravel, or WordPress, developers often encounter various error messages that can range from simple syntax issues to more complex function call errors. One such error that developers might encounter is the “Call to a member function getCollectionParentId()
on null” error. This error message can be cryptic at first, but understanding its root cause and how to resolve it can save developers significant time and frustration.
Table of Contents:
- Understanding the Error Message
- Possible Causes
- Debugging the Error
- Common Scenarios Where the Error Appears
- How to Resolve the Error
- Best Practices to Avoid the Error in the Future
- Conclusion
1. Understanding the Error Message
Before diving into potential solutions, it’s crucial to understand what this error message is trying to tell us. In PHP, an error stating “Call to a member function on null” typically means that the code is attempting to call a method (in this case, getCollectionParentId()
) on a variable or object that is, at that point in execution, null
.
PHP throws this error because you can only call methods on valid objects or instances of a class. When the object is null, PHP doesn’t know what class or method you’re referring to, and it generates this error.
Let’s break down the error:
- Call to a member function: This indicates that the program is trying to execute a method (a function tied to a class) on an object.
getCollectionParentId()
: This is the method that is being called.- On null: The object on which the method is being invoked is
null
, meaning it hasn’t been instantiated, or it has somehow lost its value before the method was called.
Thus, the root issue is that the object you’re attempting to access is null
at the time the method is called.
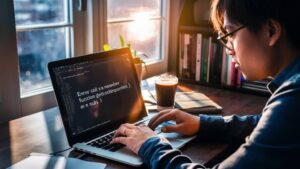
2. Possible Causes
There are several potential causes for this error, and understanding these will help in determining how to fix the issue:
2.1 Object Not Initialized
The most common cause of the error is that the object you’re working with hasn’t been properly initialized. In PHP, objects are instances of classes, and if the object you’re calling the method on is not instantiated (i.e., it hasn’t been given a value), it will be null
.
2.2 Missing or Incorrect Data in the Database
If you’re working with a content management system (CMS) like Magento, Drupal, or WordPress, this error could arise due to missing or corrupt data in the database. For instance, if you’re trying to access the parent ID of a collection and that collection doesn’t exist in the database, PHP will return null
when attempting to retrieve it.
2.3 Incorrect Method Chaining
In some cases, developers write multiple method calls in a single line of code, commonly known as method chaining. If any of the intermediate methods return null
, the subsequent method calls will throw an error. For example:
If getParent()
returns null
, then trying to call getCollectionParentId()
will result in the error.
2.4 Improper Dependency Injection
In modern PHP frameworks, dependency injection is used to pass objects around the application. If there’s an issue with dependency injection (like the required service isn’t being injected correctly), the object could be null
when you try to use it.
2.5 Incorrect Context
If you’re calling the method getCollectionParentId()
in a context where it doesn’t make sense (e.g., you’re outside the intended scope of the collection object), you might also encounter this error.
3. Debugging the Error
Once you’ve understood the possible causes, the next step is to debug the issue. Here’s how you can go about it:
3.1 Check if the Object is Null
The first thing to check is whether the object on which you’re trying to call getCollectionParentId()
is actually null
. A simple way to check this is by adding a condition before calling the method:
if ($collection !== null) {
$parentId = $collection->getCollectionParentId();
} else {
echo 'The collection object is null';
}
By doing this, you can avoid the error and see where the object becomes null
.
3.2 Use Debugging Tools
If you’re working with a modern PHP framework, you can use debugging tools like Xdebug. Xdebug allows you to set breakpoints and step through your code line by line. This makes it easier to see where the object is becoming null
.
3.3 Log the Object’s State
Another way to debug this error is by logging the state of the object just before calling the method:
var_dump($collection);
This will output the value of the $collection
object and allow you to see whether it is null
or if it has the expected data.
3.4 Check Database Integrity
If the method is supposed to retrieve data from a database, it’s worth checking whether the necessary data is present. For instance, if getCollectionParentId()
is fetching a parent ID from the database, you should ensure that the relevant entry exists.
4. Common Scenarios Where the Error Appears
There are several scenarios in which this error might occur, especially in PHP-based content management systems or frameworks. Below are a few common scenarios:
4.1 Magento
In Magento, this error might occur if you’re working with product categories or collections and trying to access a parent collection that doesn’t exist or hasn’t been properly initialized.
Example:
$category = $this->categoryRepository->get($categoryId);
$parentId = $category->getParentCategory()->getId();
If getParentCategory()
returns null
, this will lead to the “Call to a member function” error.
4.2 Laravel
In Laravel, this error might appear if you’re working with relationships between models, such as trying to access a parent or child relationship when the related model doesn’t exist in the database.
Example:
$post = Post::find($postId);
$parentId = $post->category->parent->id;
If either category
or parent
is null
, this will trigger the error.
4.3 WordPress
In WordPress, this error might occur when working with custom post types or taxonomies. If you’re trying to retrieve a parent term for a taxonomy that doesn’t have a parent, or the term itself is null
, you’ll encounter this error.
5. How to Resolve the Error
Once you’ve identified the root cause, resolving the error becomes easier. Below are some strategies:
5.1 Ensure Proper Object Initialization
Before calling getCollectionParentId()
, make sure that the object is properly initialized. This might involve fetching the object from the database or ensuring that the necessary dependency is injected.
if ($collection) {
$parentId = $collection->getCollectionParentId();
} else {
// Initialize the collection or handle the null case
}
5.2 Check for Database Integrity
If the error is related to missing or corrupt data in the database, you may need to fix the data issue. This could involve adding the missing parent ID in the database or ensuring that the collection you’re trying to access actually exists.
5.3 Use Safe Method Calls
In PHP, you can use the null coalescing operator (??
) to provide a default value if the object is null
:
$parentId = $collection->getCollectionParentId() ?? 'default-value';
This ensures that even if the object is null
, the program won’t crash.
5.4 Refactor Method Chaining
If the error is caused by method chaining, you can break the chain and check each object individually:
$parent = $this->getCollection()->getParent();
if ($parent) {
$parentId = $parent->getCollectionParentId();
}
5.5 Handle Dependency Injection Issues
If the error stems from dependency injection problems, make sure that the required service or object is being injected properly into your class. This might involve checking your service configuration or refactoring your code to ensure that the necessary dependencies are available when the method is called.
6. Best Practices to Avoid the Error in the Future
6.1 Defensive Programming
Practice defensive programming by always checking for null
values before calling methods. This might seem like overkill, but it can prevent many runtime errors.
6.2 Proper Error Handling
In larger applications, make sure you have proper error handling mechanisms in place. Catching exceptions and logging errors can help you diagnose problems more quickly.
6.3 Database Validation
Ensure that your database is consistent and that relationships between objects (like parent-child relationships) are properly maintained. You can implement database constraints to enforce this.
6.4 Use Static Analysis Tools
Tools like PHPStan or Psalm can analyze your PHP code for potential issues like calling methods on null
objects. These tools can catch such errors before they happen in production.
7. Conclusion
The “Call to a member function getCollectionParentId()
on null” error can be frustrating, but with the right approach to debugging and problem-solving, it’s usually